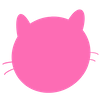
CLASS WEB
PinkCoding
- web
- python
- swift
- dart
- java
Scratch Web
PLAY
<!doctype html> <html> <head> <title>HELLO WORLD</title> <script type="text/javascript" src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> window.onload = function(){ document.getElementsByTagName('h1')[0].style.fontSize = '35px'; } </script> <style> *{margin:0;padding:0} html{background:#000;padding:40px} h1{color:skyblue;margin-top:40px} h1:nth-child(3){ font-size:130px;color:hotpink; font-weight:bold;text-transform:uppercase } </style> </head> <body> <h1>Welcome</h1> <h1>to</h1> <h1>PinkCoding</h1> </body> </html>
Scratch Python
PLAY
def plus(n1 = 0, n2 = 0): return n1 + n2; print(plus(5,10));
Scratch Swift
PLAY
func plus(int1: Int, int2: Int) -> Int { return int1 + int2 } print(plus(int1: 15, int2: 10))
Scratch Dart
PLAY
void main() { int plus(int n, int i){ return n + i; } print(plus(10, 34)); }
Scratch Java
PLAY
public class AddNumbers { public static int add(int a, int b) { return a + b; } public static void main(String[] args) { int num1 = 22; int num2 = 45; int result = add(num1, num2); System.out.println(result); } }